If you're on the latest (8) version of Ruby on Rails, there's a nice shortcut to add the not null modifier to your database columns. Just add an exclamation mark after the type, and Rails will mark that column as not null.
For example, consider the following generator command.
$ bin/rails generate migration CreateUsers email_address:string!:uniq password_digest:string!
It will produce the following migration.
class CreateUsers < ActiveRecord::Migration[8.0]
def change
create_table :users do |t|
t.string :email_address, null: false
t.string :password_digest, null: false
t.timestamps
end
add_index :users, :email_address, unique: true
end
end
Pretty handy!
P.S. While reading the corresponding pull request from DHH, I came across the starts_ends_with.rb
core extension, which simply extends the String class by aliasing starts_with?
and ends_with?
to Ruby's built-in start_with?
and end_with?
methods, which always felt a bit awkward to me while reading.
# frozen_string_literal: true
class String
alias :starts_with? :start_with?
alias :ends_with? :end_with?
end
Love the lengths Rails will go to make the code read like prose.
One more thing: I also liked how he used intermediate variables to clearly show what the method is returning. The first version doesn't immediately tell you the type of returned values, whereas the second option makes it obvious.
# From
return $1, size: $2.to_sym
# To
parsed_type, parsed_options = $1, { size: $2.to_sym }
...
...
return parsed_type, parsed_options
A nice illustration of Martin Fowler's Extract Variable refactoring. Check out my notes on Refactoring to learn more. Highly recommend reading this book.
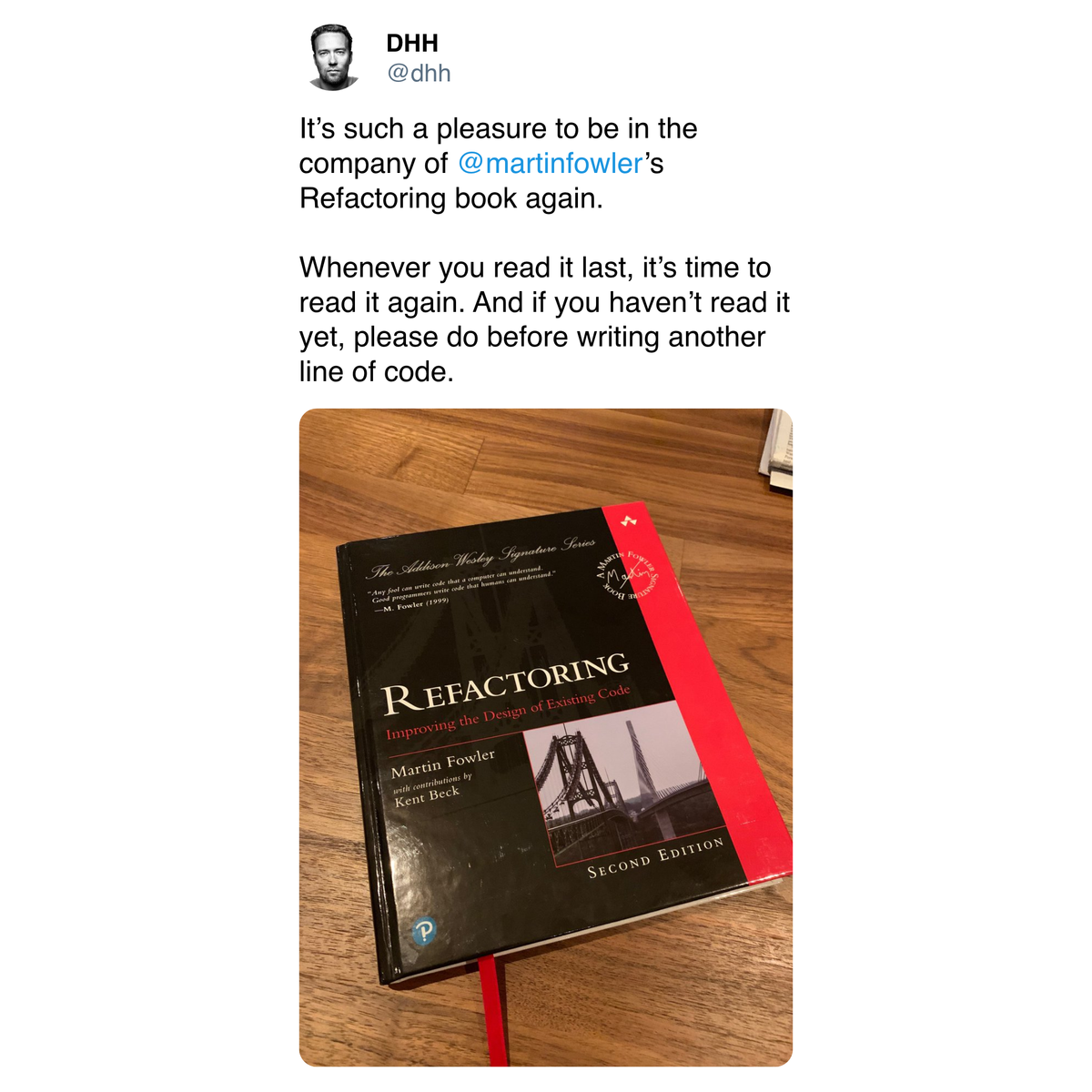
That's a wrap. I hope you found this article helpful and you learned something new.
As always, if you have any questions or feedback, didn't understand something, or found a mistake, please leave a comment below or send me an email. I reply to all emails I get from developers, and I look forward to hearing from you.
If you'd like to receive future articles directly in your email, please subscribe to my blog. Your email is respected, never shared, rented, sold or spammed. If you're already a subscriber, thank you.