Everything in Ruby is an object. The Ruby interpreter runs every line of code you write in the context of an object. The self
keyword points to this object.
Here are the four important rules to keep in mind with the self
keyword:
- Only one object can be
self
at a given time. self
is constantly changing as a program executes.- When you call a method, the receiver of that method (i.e. the object on which you called the method) becomes
self
. - All methods without an explicit receiver are called on
self
.
Here are the different forms self
can take, depending on where it's used.
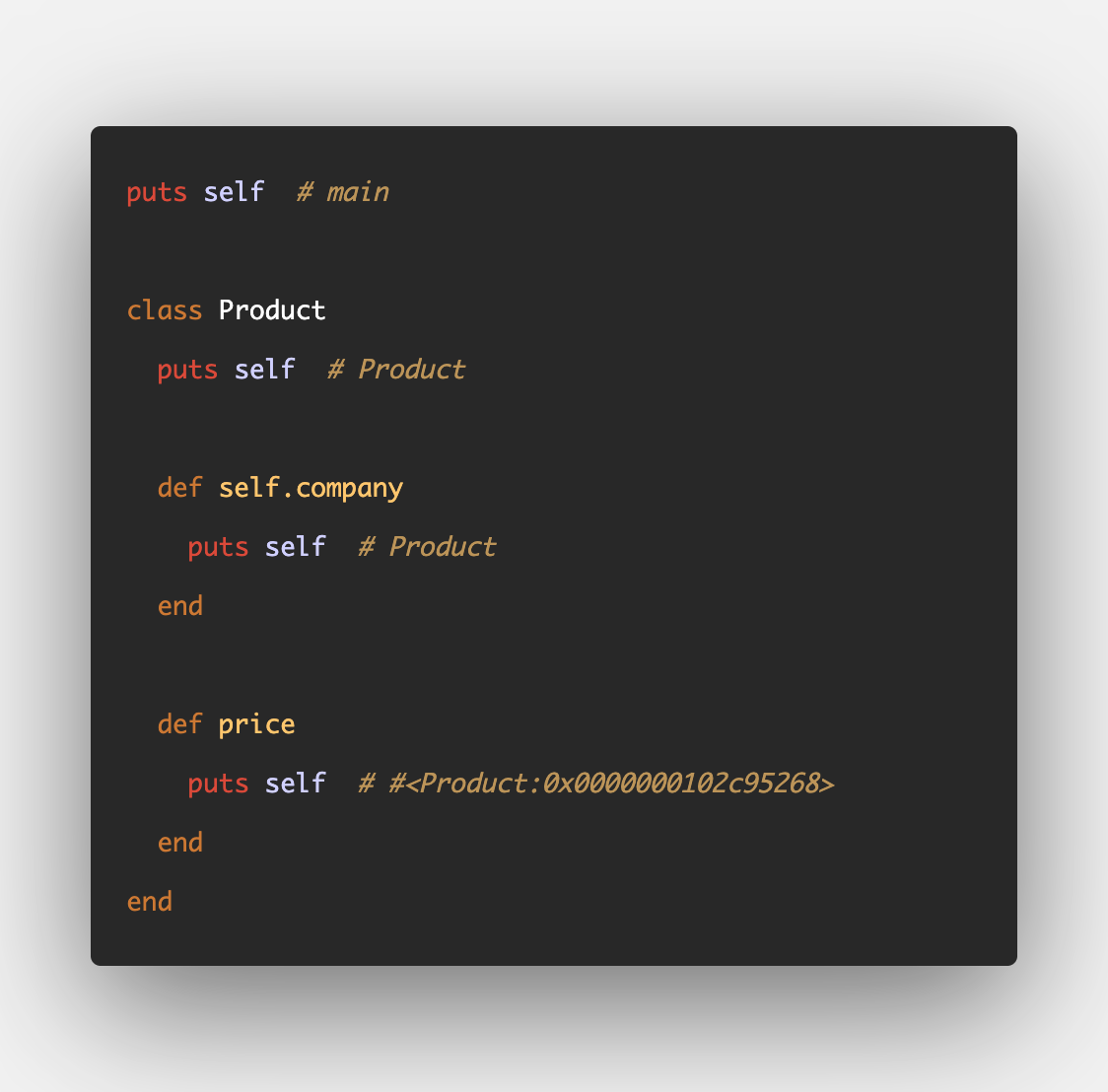
Top-Level
At the top level, self
points to the main
object, which is an instance of Object
class.
As soon as a Ruby program starts, the Ruby interpreter creates an object called main
, and all subsequent code is executed in the context of this object.
puts self # main
puts self.class # Object
This context is also called top-level context.
Inside a Class or Module
Ruby lets you run any code inside the class or module definition, where the role of self
is taken by the class or module itself.
class Language
puts self # Language
end
module Maths
puts self # Maths
end
Inside a Class Method
The class method runs in the context of the class, not its instance. The class itself 'owns' that method.
In a class method, self
points to the class.
class Language
def self.run
puts self # Language
end
end
It works similarly for a module's class methods.
module Maths
def self.calculate
puts self # Maths
end
end
Inside an Instance Method
All instance methods belong to the instance. Hence, self
points to the specific instance of the class.
class Language
def compile
puts self # #<Language:0x00007fc7c191c9f0>
end
end
ruby = Language.new
ruby.compile
When you call a method, Ruby looks up the method in the object's ancestor chain and then executes the method with the receiver as self
.
Inside a Module (Mixin) Method
Modules are one of Ruby's coolest features. They're somewhat similar to classes: they hold methods. However, you can't instantiate them directly. Modules let you mix these methods into another class (hence the word mixin💡).
When you include a module into a class, i.e. (mix in the module's methods as instance methods), the self
object in that method points to the instance of that class.
module Task
def execute
puts self # #<Project:0x0000000107fdce30>
end
end
class Project
include Task
end
p = Project.new
p.execute
When you extend a module into a class, i.e. (mix in the module's methods as class methods), the self
object in that method points to the class.
module Task
def execute
puts self # Client
end
end
class Client
extend Task
end
Client.execute
So that was a brief overview of how the self
keyword works in Ruby. Understanding it will give you new powers to wield in your Rails apps.
I hope you found this article useful and that you learned something new. If you have any questions or feedback, please send me an email. I look forward to hearing from you.
Please subscribe to my blog below if you'd like to receive future articles directly in your email. If you're already a subscriber, THANK YOU 🙏