Sometimes, you need a config-like property on a class, such as a logger. Rails provides the Configurable
concern that lets you accomplish this. It provides a config
method that allows you to access (read and write) configuration options using the method-like syntax.
class Invoice
include ActiveSupport::Configurable
config_accessor :type
end
Invoice.type # => nil
Invoice.type = :cheque
Invoice.type # => :cheque
A nice benefit of using Configurable
is that all the instances get the same config option, which they can override independently without affecting the class config.
invoice = Invoice.new
invoice.type # => :cheque
invoice.type = :credit
invoice.type # => :credit
Invoice.type # => :cheque
new_invoice = Invoice.new
new_invoice.type # => :cheque
Behind the scenes, the config
method returns an instance of OrderedOptions
class. To learn more, check out my article on ordered options.
Practical Use
Rails uses the Configurable
concern to add logger
configuration option on the controllers.
The AbstractController::Base
class includes this concern so you can configure a logger on the controllers.
# actionpack/lib/abstract_controller/base.rb
require "active_support/configurable"
module AbstractController
class Base
include ActiveSupport::Configurable
# rest of the code
end
end
end
Then the AbstractController::Logger
module (concern) configures the :logger
config on the controllers.
# actionpack/lib/abstract_controller/logger.rb
module AbstractController
module Logger
extend ActiveSupport::Concern
included do
config_accessor :logger
end
end
end
This allows you to configure loggers on your controllers, as well as access them whenever needed.
To learn more about concerns and how they work, check out this detailed article.
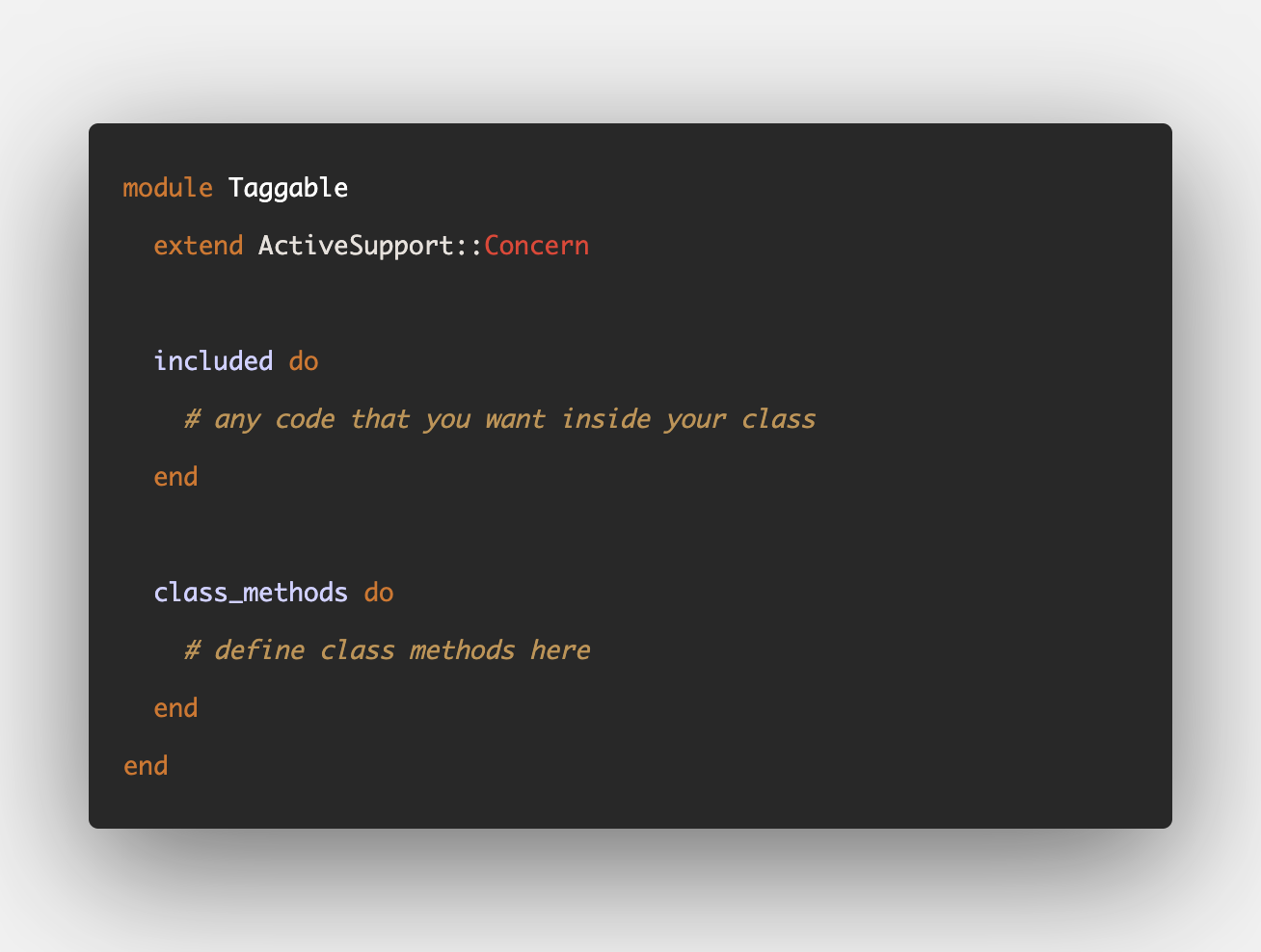
I hope you found this article useful and that you learned something new.
If you have any questions or feedback, didn't understand something, or found a mistake, please leave a comment below or send me an email. I look forward to hearing from you.
Please subscribe to my blog if you'd like to receive future articles directly in your email. If you're already a subscriber, thank you.